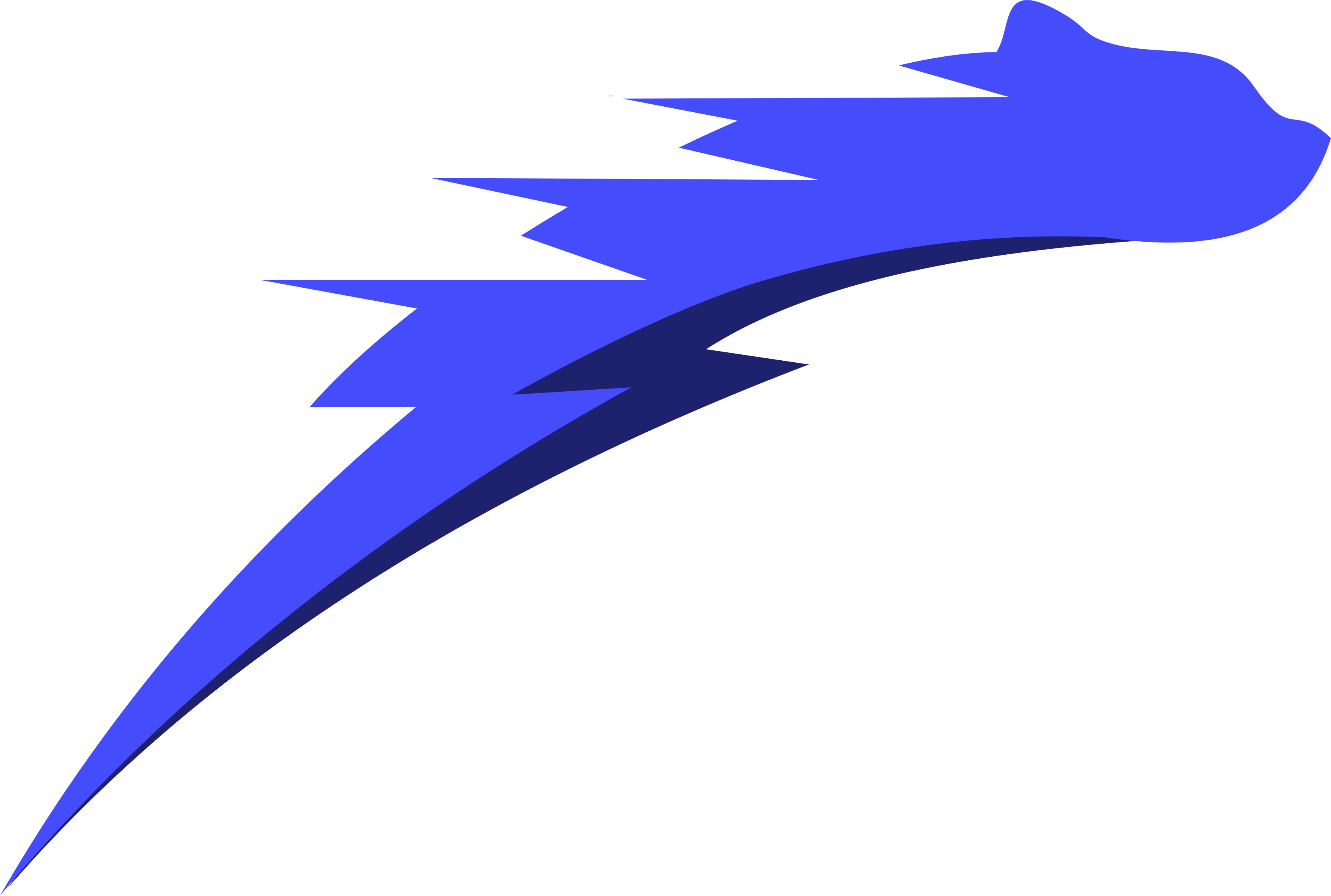
This section covers how to register a user account. This account (after a successful creation), will be used to place orders and every other request a user can do on the official mobile application
Here are the requirements:
Field | Sample Value | Required | Description |
---|---|---|---|
full_name | John Doe | YES | The new user's full name |
phone_number | 08012345678 | YES | The new user's local phone number (without the dialing code). Accepted values can be 08012345678 or without the leading zero (0), as in 8012345678 |
dialing_code | +234 | YES | Accepted values are: +93, +358, +355, +213, +1684, +376, +244, +1264, +672, +1268, +54, +374, +297, +61, +43, +994, +1242, +973, +880, +1246, +375, +32, +501, +229, +1441, +975, +591, +387, +267, +47, +55, +246, +673, +359, +226, +257, +855, +237, +1, +238, +345, +236, +235, +56, +86, +61, +61, +57, +269, +242, +243, +682, +506, +225, +385, +53, +357, +420, +45, +253, +1767, +1849, +593, +20, +503, +240, +291, +372, +251, +500, +298, +679, +358, +33, +594, +689, +262, +241, +220, +995, +49, +233, +350, +30, +299, +1473, +590, +1671, +502, +44, +224, +245, +592, +509, +672, +379, +504, +852, +36, +354, +91, +62, +98, +964, +353, +44, +972, +39, +1876, +81, +44, +962, +7, +254, +686, +850, +82, +383, +965, +996, +856, +371, +961, +266, +231, +218, +423, +370, +352, +853, +389, +261, +265, +60, +960, +223, +356, +692, +596, +222, +230, +262, +52, +691, +373, +377, +976, +382, +1664, +212, +258, +95, +264, +674, +977, +31, +599, +687, +64, +505, +227, +234, +683, +672, +1670, +47, +968, +92, +680, +970, +507, +675, +595, +51, +63, +64, +48, +351, +1939, +974, +40, +7, +250, +262, +590, +290, +1869, +1758, +590, +508, +1784, +685, +378, +239, +966, +221, +381, +248, +232, +65, +421, +386, +677, +252, +27, +211, +500, +34, +94, +249, +597, +47, +268, +46, +41, +963, +886, +992, +255, +66, +670, +228, +690, +676, +1868, +216, +90, +993, +1649, +688, +256, +380, +971, +44, +1, +598, +998, +678, +58, +84, +1284, +1340, +681, +967, +260, +263 |
sampleemail@domain.com | NO | User's email | |
referrer | abcdef | NO | Referral code of another user who referred the current user |
Here is a sample API CALL
curl
--location --request POST
"https://api.fastryders.com/user-mgt/register-user"
--header
"x-fastryders-token: FRYD-TEST-P2mJBDwqgbgJDM0lOOEp7Gv5cxDzycgJb0P90AS7"
--header
"Authorization: Basic c2FtcGxlQGVtYWlsLmNvbTp5b3VyIHBhc3N3b3Jk"
--form
"full_name=John Doe"
--form
"phone_number=08012345678"
--form
"dialing_code=234"
Response should be in JSON. Response with status error, should have message with description of the error
Here's is a typical success response expressed in a table with description
Key | Sample value | Description | ||||
---|---|---|---|---|---|---|
status | success | Account created successfully if LIVE TOKEN was passed | ||||
message | User account created successfully - authentication code sent to supplied phone number | Request description | ||||
data |
{
"app_token":"00000000000000000000000000000000000000000000000000", "phone": "2348012345678" } |
|
If for any reason you decide to request for a new verification code like the one sent after user account registration, then this section covers how to do that.
Here are the requirements:
Field | Sample Value | Required | Description |
---|---|---|---|
phone_number | 2348012345678 | YES | Phone number that was returned when account was created |
Here is a sample API CALL
curl
--location --request POST
"https://api.fastryders.com/user-mgt/resend-auth-otp"
--header
"x-fastryders-token: FRYD-TEST-P2mJBDwqgbgJDM0lOOEp7Gv5cxDzycgJb0P90AS7"
--header
"Authorization: Basic c2FtcGxlQGVtYWlsLmNvbTp5b3VyIHBhc3N3b3Jk"
--form
"phone_number=2348012345678"
Here's is a typical success response expressed in a table with description
Key | Sample value | Description |
---|---|---|
status | success | OTP was sent successfully |
message | OTP sent | Request description |
This section covers how to activate a registered account.
Every account registered is first passive and can't be used for any transaction unless activated.
We do this to ensure a basic level of commitment from the user.
Upon a successful registration, an OTP is sent to the registered phone number (and the registered email address if supplied).
Supply this OTP to get your accounts activated. To do this,
Field | Sample Value | Required | Description |
---|---|---|---|
phone_number | 2348012345678 | YES | Phone number that was received (as phone field) upon successful account creation |
otp | 1234 | YES | The authentication code that was sent to the registered phone number upon successful account creation. For Test Transactions, use any digit |
Here is a sample API CALL
curl
--location --request POST
"https://api.fastryders.com/user-mgt/activate-user"
--header
"x-fastryders-token: FRYD-TEST-P2mJBDwqgbgJDM0lOOEp7Gv5cxDzycgJb0P90AS7"
--header
"Authorization: Basic c2FtcGxlQGVtYWlsLmNvbTp5b3VyIHBhc3N3b3Jk"
--form
"phone_number=2348012345678"
--form
"otp=1234"
Here's is a typical success response expressed in a table with description
Key | Sample value | Description |
---|---|---|
status | success | Verification was successful |
message | Verification was successful | Request description |
data |
{ "app_token": "bgJDM0lOOEp7Gv5cxDzycgJb0P90AS7" } |
This sction covers how to verify if a phone number is registered
Here are the requirements:
Field | Sample Value | Required | Description |
---|---|---|---|
phone_number | 2348012345678 | YES | Phone number in the format country_code and phone number without leading zero |
Here is a sample API CALL
curl
--location --request POST
"https://api.fastryders.com/user-mgt/resend-auth-otp"
--header
"x-fastryders-token: FRYD-TEST-P2mJBDwqgbgJDM0lOOEp7Gv5cxDzycgJb0P90AS7"
--header
"Authorization: Basic c2FtcGxlQGVtYWlsLmNvbTp5b3VyIHBhc3N3b3Jk"
--form
"phone_number=2348012345678"
Here's is a typical success response expressed in a table with description
Key | Sample value | Description |
---|---|---|
status | success | Request was successful |
message | Invalid phone | Phone number is invalid |
data | Invalid phone |
{ "phone_status": "INVALID_PHONE" } |